Користуємось стандартною бібліотекою "google.golang.org/api/sheets/v4"
**Список прикладів:**
* Розмічаємо таблицю щоб її було видно (border)
* Виділяємо шапку
* Фіксуємо шапку
* Додаємо фільтр до шапки
* Встановлюємо формат колонки дата з випадаючим календарем
* Встановлюємо формат колонки з випадаючими варіантами на вибір
**Розмічаємо таблицю щоб її було видно (border):**
```
requests := []*sheets.Request{}
requests = append(requests, &sheets.Request{
UpdateBorders: &sheets.UpdateBordersRequest{
Range: &sheets.GridRange{
SheetId: 0, StartRowIndex: 0, EndRowIndex: 999, EndColumnIndex: 6,
},
Top: &sheets.Border{
Style: "SOLID", Width: 1, Color: &sheets.Color{Alpha: 1.0},
},
Bottom: &sheets.Border{
Style: "SOLID", Width: 1, Color: &sheets.Color{Alpha: 1.0},
},
InnerHorizontal: &sheets.Border{
Style: "SOLID", Width: 1, Color: &sheets.Color{Alpha: 1.0},
},
InnerVertical: &sheets.Border{
Style: "SOLID", Width: 1, Color: &sheets.Color{Alpha: 1.0},
},
Right: &sheets.Border{
Style: "SOLID", Width: 1, Color: &sheets.Color{Alpha: 1.0},
},
},
},
)
```
**Виділяємо шапку:**
```
requests = append(requests, &sheets.Request{
RepeatCell: &sheets.RepeatCellRequest{
Range: &sheets.GridRange{
SheetId: 0, StartRowIndex: 0, EndRowIndex: 1, EndColumnIndex: 6,
},
Cell: &sheets.CellData{
UserEnteredFormat: &sheets.CellFormat{
BackgroundColor: &sheets.Color{Red: 43.0, Green: 43.0, Blue: 43.0}, // Background шапки
HorizontalAlignment: "CENTER",
TextFormat: &sheets.TextFormat{
ForegroundColor: &sheets.Color{Red: 0.0, Green: 0.0, Blue: 204.0}, FontSize: 13, Bold: true}}}, // Текст Шапки
Fields: "userEnteredFormat(backgroundColor,textFormat,horizontalAlignment)",
},
},
)
```
**Фіксуємо шапку:**
```
requests = append(requests, &sheets.Request{
UpdateSheetProperties: &sheets.UpdateSheetPropertiesRequest{
Properties: &sheets.SheetProperties{SheetId: 0, GridProperties: &sheets.GridProperties{FrozenRowCount: 1}},
Fields: "gridProperties.frozenRowCount",
},
},
)
```
**Додаємо фільтр до шапки:**
```
requests = append(requests, &sheets.Request{
SetBasicFilter: &sheets.SetBasicFilterRequest{Filter: &sheets.BasicFilter{
Range: &sheets.GridRange{
SheetId: 0, StartRowIndex: 0, EndRowIndex: 1, StartColumnIndex: 0, EndColumnIndex: 6,
},
},
},
})
```
**Встановлюємо формат колонки дата з випадаючим календарем:**
```
requests = append(requests, &sheets.Request{
RepeatCell: &sheets.RepeatCellRequest{
Range: &sheets.GridRange{
SheetId: 0, StartRowIndex: 1, EndRowIndex: 999, StartColumnIndex: 0, EndColumnIndex: 1, // 1 колонка
},
Cell: &sheets.CellData{
UserEnteredFormat: &sheets.CellFormat{
NumberFormat: &sheets.NumberFormat{
Type: "DATE",
Pattern: "dd/mm/yy",
}}},
Fields: "userEnteredFormat.numberFormat",
},
},
)
requests = append(requests, &sheets.Request{
SetDataValidation: &sheets.SetDataValidationRequest{
Range: &sheets.GridRange{
SheetId: 0, StartRowIndex: 1, EndRowIndex: 999, StartColumnIndex: 0, EndColumnIndex: 1, // 1 колонка
},
Rule: &sheets.DataValidationRule{
Condition: &sheets.BooleanCondition{
Type: "DATE_IS_VALID",
},
ShowCustomUi: true,
},
},
},
)
```
**Встановлюємо формат колонки з випадаючими варіантами на вибір:**
```
r := []*sheets.ConditionValue{}
r = append(r, &sheets.ConditionValue{
UserEnteredValue: "NEW",
})
r = append(r, &sheets.ConditionValue{
UserEnteredValue: "IN PROGRESS",
})
r = append(r, &sheets.ConditionValue{
UserEnteredValue: "COMPLETED",
})
requests = append(requests, &sheets.Request{
SetDataValidation: &sheets.SetDataValidationRequest{
Range: &sheets.GridRange{
SheetId: 0, StartRowIndex: 1, EndRowIndex: 999, StartColumnIndex: 4, EndColumnIndex: 5, // 1 колонка
},
Rule: &sheets.DataValidationRule{
Condition: &sheets.BooleanCondition{
Type: "ONE_OF_LIST",
Values: r,
},
ShowCustomUi: true,
},
},
},
)
```
**Застосовуємо:**
```
rb := &sheets.BatchUpdateSpreadsheetRequest{
Requests: requests,
}
_, err := s.Service.Spreadsheets.BatchUpdate(spreadsheetId, rb).Do()
```
**Маємо наступний результат:**
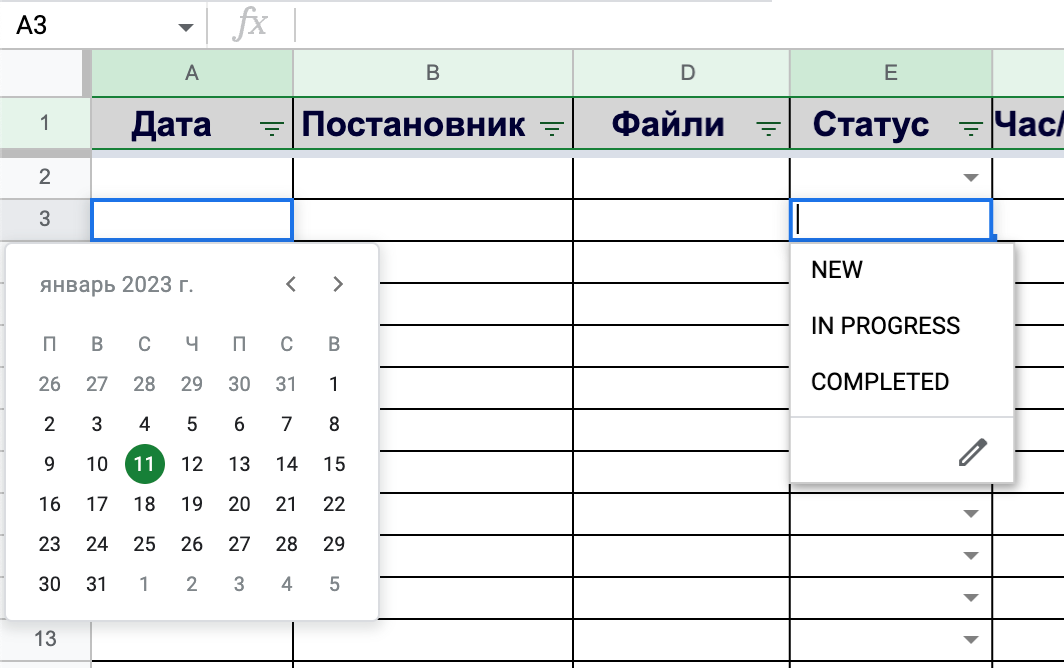